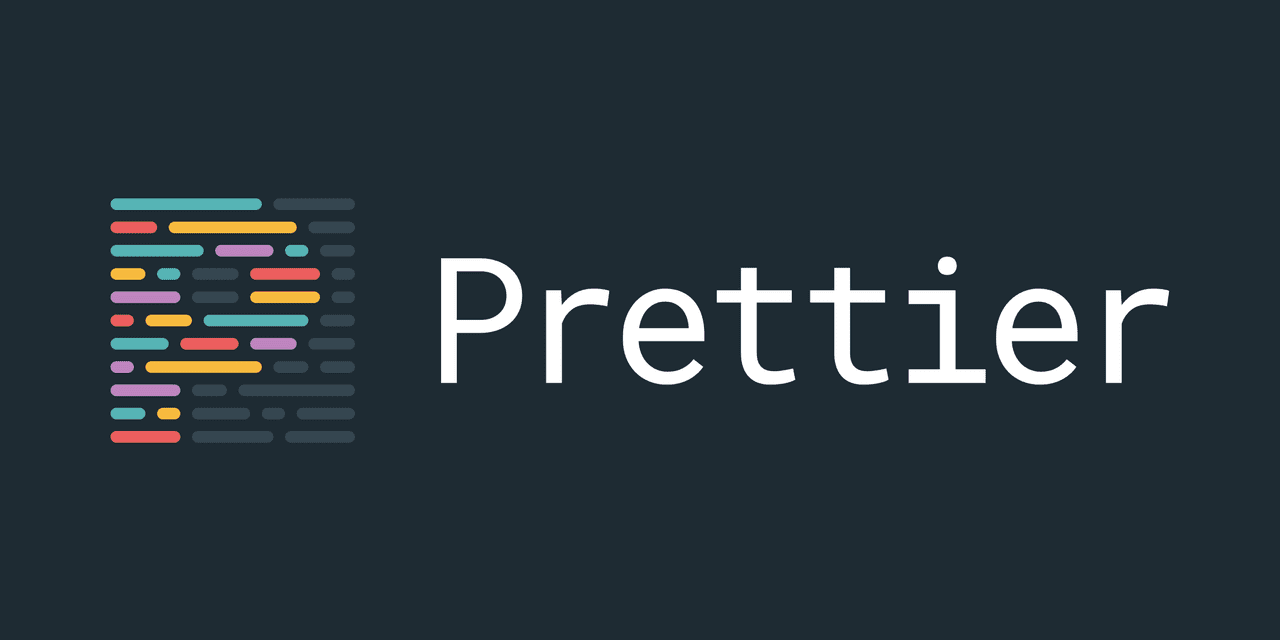
Prettier and ESLint are essential tools in any JavaScript/TypeScript project, especially for large, collaborative projects. Next.js offers built-in support for ESLint, but to maximize productivity and code cleanliness, adding Prettier is highly recommended. Let’s walk through setting up both in a Next.js project.
Step 1: Initial Project Setup
If you haven’t created your Next.js project yet, start by initializing it with:
npx create-next-app@latest
Step 2: Install ESLint and Prettier
First, install ESLint, Prettier, and some essential plugins and configurations that allow them to work seamlessly together.
npm install eslint prettier eslint-config-prettier eslint-plugin-prettier --save-dev
Here’s a quick breakdown of these packages:
eslint
: Lints your code.prettier
: Formats your code.eslint-config-prettier
: Disables ESLint rules that would conflict with Prettier.eslint-plugin-prettier
: Runs Prettier as an ESLint rule to show formatting errors.
Step 3: Configure ESLint
Next.js comes with ESLint integration, so let’s configure it for compatibility with Prettier.
In your project root, create a
.eslintrc.json
file:
{
"extends": [
"next/core-web-vitals",
"eslint:recommended",
"plugin:prettier/recommended"
],
"rules": {
"prettier/prettier": ["error"]
}
}
Explanation of .eslintrc.json
settings:
next/core-web-vitals
: Uses Next.js-recommended settings.eslint:recommended
: Sets up essential ESLint rules.plugin:prettier/recommended
: Integrates Prettier with ESLint."prettier/prettier": ["error"]
: Displays Prettier errors as ESLint errors.
Step 4: Configure Prettier
Add a Prettier configuration file to define your formatting rules. Create a .prettierrc
file in your project root:
{
"semi": true,
"trailingComma": "es5",
"singleQuote": true,
"printWidth": 80,
"tabWidth": 2
}
Customize these options based on your preferences. Here’s a brief explanation of each setting:
"semi": true
: Adds semicolons at the end of statements."trailingComma": "es5"
: Adds trailing commas where valid in ES5 (e.g., objects, arrays)."singleQuote": true
: Prefers single quotes over double quotes."printWidth": 80
: Sets a maximum line length of 80 characters."tabWidth": 2
: Sets tab width to 2 spaces.
Step 5: Configure ESLint and Prettier Ignore Files
You may want to exclude certain files and directories from being linted or formatted. Create .eslintignore
and .prettierignore
files in the root directory with the following content:
node_modules
.next
out
These files will prevent ESLint and Prettier from running on the node_modules
, .next
, and out
directories, which contain build-related files and dependencies.
Step 6: Add Formatting and Linting Scripts
In your package.json
, add scripts to simplify running ESLint and Prettier across your project.
{
"scripts": {
"lint": "eslint . --ext .js,.jsx,.ts,.tsx",
"format": "prettier --write ."
}
}
lint
: Runs ESLint on all JavaScript and TypeScript files in your project.format
: Formats your entire project using Prettier.
Step 7: Test the Setup
Run ESLint to check for linting issues:
npm run lint
ESLint should display any issues based on your configuration.
Run Prettier to format your code:
npm run format
This command will format your code according to your Prettier configuration.
Conclusion
You now have Prettier and ESLint configured for your Next.js project! This setup ensures consistent, clean code and catches potential errors early in the development process. As you grow your project or collaborate with others, these tools will help maintain high code quality effortlessly.
Happy coding!